Mastering React Components for Your Next Project in 2023
Written on
Understanding React Components
React components are fundamental building blocks for your web applications. They allow for code reuse, enabling you to write once and utilize multiple times throughout your project. This efficiency helps you focus on your work without unnecessary stress. In React, components are crucial, with developers commonly using hundreds of them to streamline their coding processes.
Types of Components
There are two primary types of components in React:
- Functional Component
- Class Component
Exploring Functional Components
Functional components are straightforward functions that return values when called. They simplify your code, making it more readable and maintainable. For instance:
function Name() {
return <h2>Hello, I am Nitin!</h2>;
}
This example demonstrates a Functional Component. Isn't that simple? React.js is not only easy to learn but also incredibly powerful.
To try it out, open your code editor, such as codesandbox.io or codepen, and paste the following code into "App.js":
import React from 'react';
function App() {
return <h2>Hello, I am Nitin!</h2>;
}
export default App;
Output Example
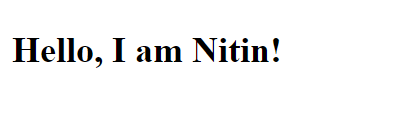
If you're puzzled about why we export the App component, here's a brief explanation. In the "index.js" file, you will find:
import { StrictMode } from "react";
import ReactDOM from "react-dom";
import App from "./App";
ReactDOM.render(
<StrictMode>
<App /></StrictMode>,
document.getElementById('root')
);
You can ignore the StrictMode for now; it's not essential. The core point is that we export the App from App.js and import it in index.js.
Components: Props and States
Components can utilize props and states, which make them dynamic and engaging. Let's clarify these concepts:
- Props: These are used to pass properties to components.
- States: These maintain the component's internal data and can change over time.
Props are immutable, while states are mutable, and states require a constructor, unlike props.
#### Utilizing Props in Functional Components
Here's how to implement props in a Functional Component:
import React from 'react';
function Hello(props) {
return <h1>Learn {props.name}</h1>;
}
export default function App() {
return (
<div>
<Hello name="React" />
<Hello name="React Native" />
</div>
);
}
In this code, we define two functions within the App function and pass props to the Hello component.
Output Example
Output from the above code will yield:
Learn React
Learn React Native
Understanding State in Functional Components
Functional components do not hold state directly; instead, they utilize Hooks. You can explore more about Hooks through this link:
State Management Using React Hooks in React Native
With React Hooks, we can manage state and other React features without needing a Class…
Class Components Explained
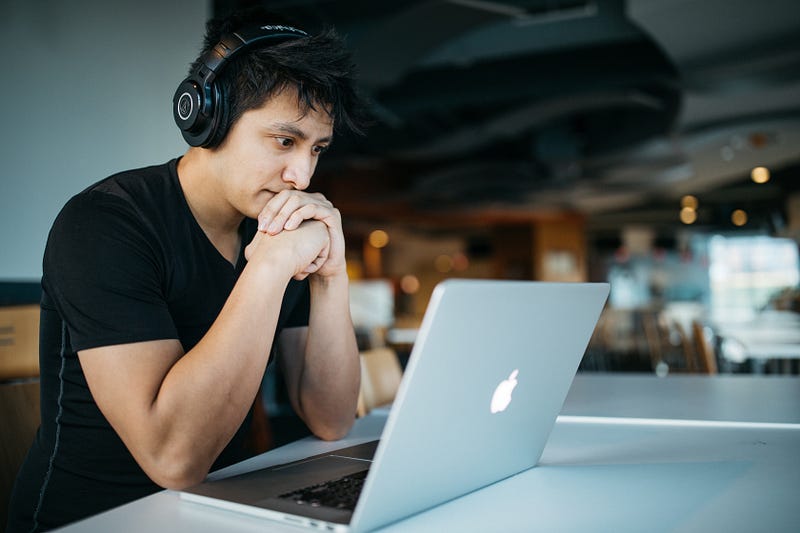
Class components resemble Functional Components but include some distinctions. The key differences are:
- Class components manage state while Functional components utilize Hooks.
- A constructor is present in Class components, absent in Functional ones.
- Class components have a render() method, which is not found in Functional Components.
For instance:
import React from 'react';
class Car extends React.Component {
render() {
return <h2>Learn React Native Basics!</h2>;}
}
export default Car;
Here, we create a Class Component by extending React.Component and implementing a render method.
Using Props in Class Components
Just like in Functional Components, you can pass props to Class Components:
import React from 'react';
import ReactDOM from 'react-dom';
class Name extends React.Component {
render() {
return <h2>I am {this.props.name}</h2>;}
}
ReactDOM.render(
<Name name="Nitin"/>,
document.getElementById('root')
);
Using State in Class Components
To manage state in Class Components, you need to use a constructor. Here's an example from React's documentation:
class Example extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0};
}
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button
onClick={() =>
this.setState({ count: this.state.count + 1 })}>
Click me
</button>
</div>
);
}
}
In this example, we initialize the count to zero in the constructor. When the button is clicked, the count increments by one.
Conclusion
Now that you have a foundational understanding of React components, props, and states, it's time to dive deeper into the documentation and practice your skills. If you appreciate my work, consider buying me a cup of coffee!
The first video titled "React or Next.js: What to Learn in 2023" provides insights into deciding between these two popular frameworks based on various questions received from the audience.
The second video, "React Native Tutorial for Beginners - Build a React Native App", offers a comprehensive guide for beginners looking to develop their first React Native application.