Mastering Async Operations with React Hooks: A Comprehensive Guide
Written on
Chapter 1: Understanding State Management in React
In traditional class-based React components, developers can utilize a callback function as the second argument of setState to execute code when the state changes. However, with the introduction of React hooks, the setState method has been replaced. Now, we employ state updater functions derived from the useState hook to manage state. This shift necessitates new strategies for executing code after a state update.
To illustrate this, we will explore how to execute asynchronous code following state changes in function components that leverage React hooks.
Section 1.1: Utilizing the useEffect Hook
The useEffect hook empowers developers to handle side effects within their components. This hook allows for the execution of side effects triggered by changes in one or more state variables. To determine which state to monitor, an array containing the states of interest is supplied as the second argument to useEffect. Consequently, any code placed in the callback function of useEffect will execute whenever the monitored state changes.
For example, consider the following implementation:
import React, { useEffect, useState } from "react";
export default function App() {
const [loading, setLoading] = useState(false);
const [loading2, setLoading2] = useState(false);
useEffect(() => {
setTimeout(() => setLoading(true), 1000);}, []);
useEffect(() => {
if (loading) {
setTimeout(() => setLoading2(true), 1000);}
}, [loading]);
return (
<>
<div>{loading.toString()}</div>
<div>{loading2.toString()}</div>
</>
);
}
In this example, we define two states: loading and loading2, which are modified within the useEffect callbacks. The first useEffect sets a timeout to update loading to true after 1000 milliseconds. The second useEffect monitors the loading state; when it turns true, it triggers another timeout to set loading2 to true after 1000 milliseconds.
Subsection 1.1.1: Visual Representation
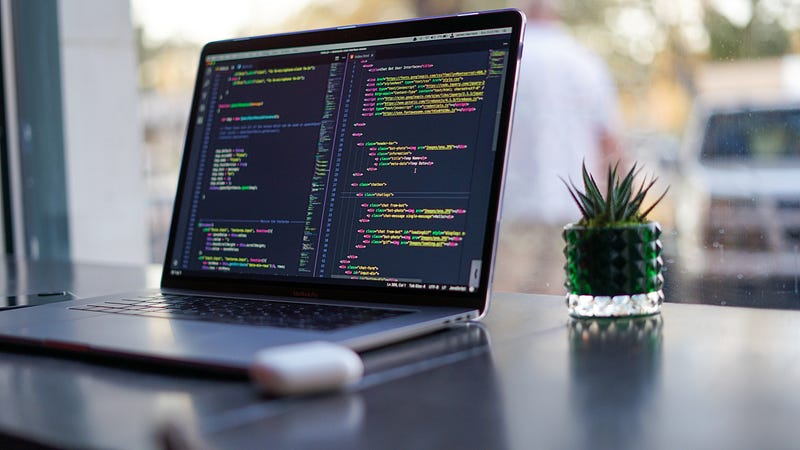
Section 1.2: The Power of useEffect in Action
By utilizing useEffect, we can seamlessly run code in response to state changes. As demonstrated, the JSX renders the values of each state, allowing us to observe them transitioning to true sequentially.
Chapter 2: Deep Dive into Asynchronous Code Execution
As we conclude, it’s evident that we can effectively manage asynchronous operations following state changes by employing the useEffect hook. By passing the states we wish to observe into the array of the second argument, we can execute code based on changes in state values within the useEffect callback.
To further enhance your understanding, consider watching the following informative videos:
This first video titled "Exploring the Async Behavior of React's useState Hook" delves into the intricacies of asynchronous behavior in React's state management.
The second video, "UseState: Asynchronous or what?" explores the nuances of the useState hook, providing valuable insights into its asynchronous nature.